Understanding PHP URL Encryption: A Comprehensive Guide
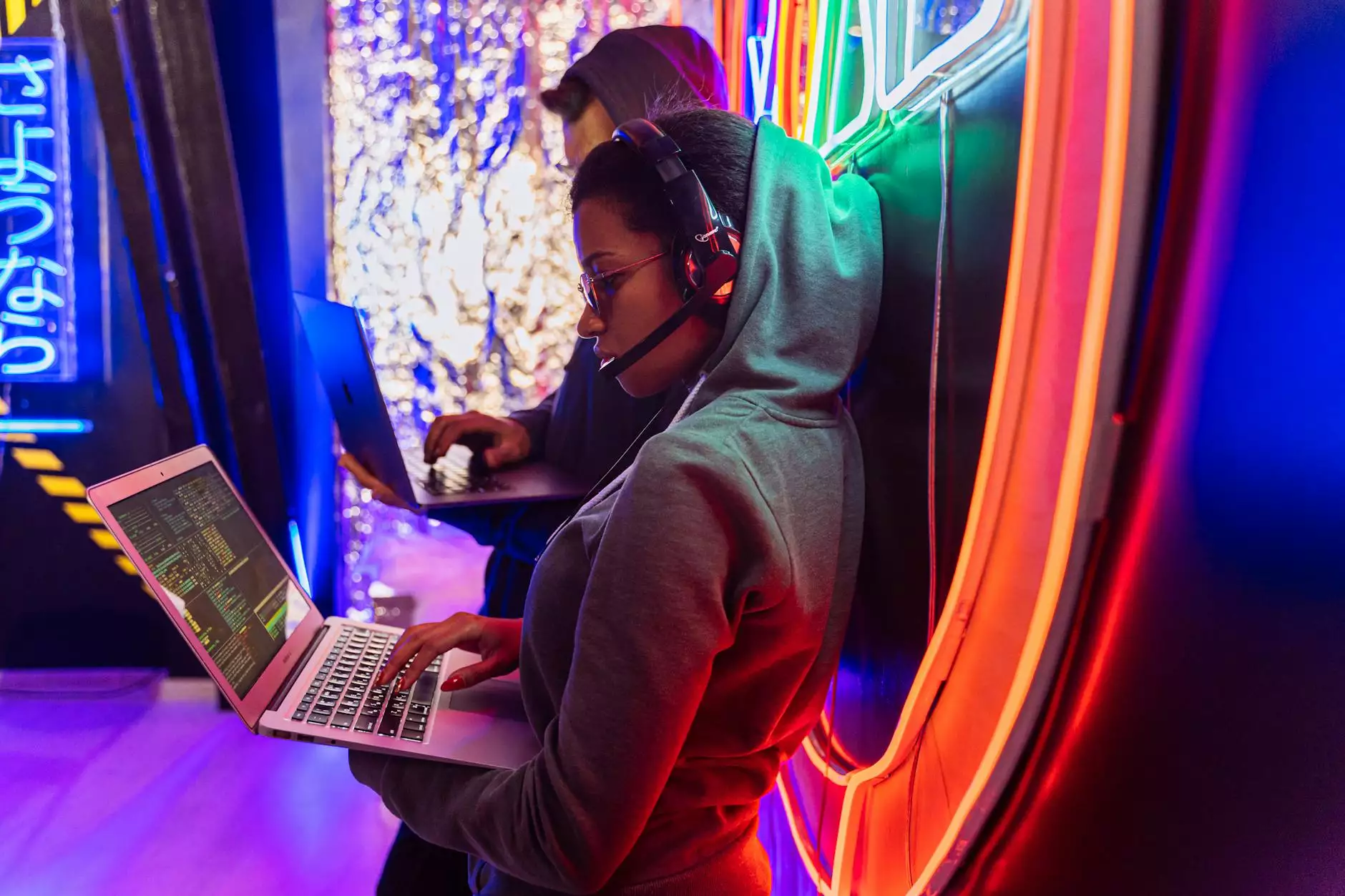
In the modern digital landscape, security is of utmost importance. As businesses, especially those in Web Design and Software Development, increasingly rely on online platforms, safeguarding sensitive data has become critical. One significant yet often overlooked aspect of web security is URL encryption. This article delves into PHP URL encryption examples to help you understand how to protect your URLs effectively.
What is URL Encryption?
URL encryption is the process of obfuscating the parameters in a URL to protect sensitive information from being read or tampered with during transmission. This practice is essential for web applications where user data, authentication tokens, and other confidential information may be exposed in the URL.
Why is URL Encryption Important?
Encryption plays a vital role in web security; here are several reasons why URL encryption is critical:
- Data Protection: Encrypting URLs helps in securing sensitive data, such as user identification or session tokens, from malicious actors.
- Integrity: It ensures that the data in the URL cannot be modified without detection.
- User Trust: Users are more likely to trust websites that take significant measures to protect their data.
- Compliance: Many industries are required to follow strict guidelines regarding data protection, making encryption a necessity.
How Does URL Encryption Work?
URL encryption typically involves using algorithms to encode the data within the URL. This data can then be safely transmitted over the web. In PHP, encryption can be accomplished using various methods, including the use of built-in functions such as base64_encode(), openssl_encrypt(), and custom algorithms.
PHP URL Encryption Examples
Below, we present a detailed example of how to encrypt and decrypt URLs in PHP. This guide will walk you through basic yet effective methods to secure your URL parameters.
Example 1: Using Base64 Encoding
Base64 encoding is a straightforward method for URL encoding. However, it should be noted that while it obscures the data, it does not provide strong encryption.
function encrypt_url($string) { return base64_encode($string); } function decrypt_url($string) { return base64_decode($string); } // Example usage $original_string = "sensitiveData=12345&userID=67890"; $encrypted_string = encrypt_url($original_string); $decrypted_string = decrypt_url($encrypted_string); echo "Original: $original_string"; echo "Encrypted: $encrypted_string"; echo "Decrypted: $decrypted_string";In this example, we create two functions: encrypt_url() and decrypt_url(). The original string is encoded using base64_encode(), and we can easily retrieve the original string by decoding it.
Example 2: Using OpenSSL for Stronger Encryption
Base64 encoding may not be sufficient for highly sensitive information. For that, using the OpenSSL library can provide robust encryption capabilities.
function encrypt($data, $key) { $iv = openssl_random_pseudo_bytes(openssl_cipher_iv_length('aes-256-cbc')); $encrypted = openssl_encrypt($data, 'aes-256-cbc', $key, 0, $iv); return base64_encode($encrypted . '::' . $iv); } function decrypt($data, $key) { list($encrypted_data, $iv) = explode('::', base64_decode($data), 2); return openssl_decrypt($encrypted_data, 'aes-256-cbc', $key, 0, $iv); } // Example usage $key = 'your-32-character-long-key'; // Secure your key $sensitive_data = "sensitiveData=12345&userID=67890"; $encrypted_data = encrypt($sensitive_data, $key); $decrypted_data = decrypt($encrypted_data, $key); echo "Original: $sensitive_data"; echo "Encrypted: $encrypted_data"; echo "Decrypted: $decrypted_data";This example demonstrates how to use OpenSSL for encryption. Here, we generate an initialization vector (IV) for added security and combine it with the encrypted data to ensure both can be used for decryption.
Best Practices for URL Encryption
While encryption is crucial, following best practices maximizes your web application's security:
- Use Secure Algorithms: Always select strong encryption algorithms like AES for securing data.
- Keep Your Keys Safe: Never hard-code your encryption keys in your source code. Instead, use environment variables or secure vaults.
- Limit Sensitive Data in URLs: Try to minimize the presence of sensitive data in URLs; use session IDs or POST methods instead.
- Regularly Update Your Security Practices: Security is an ongoing process. Regularly review and update your encryption methods.
Conclusion
In conclusion, understanding URL encryption is critical for any business operating in today's digital environment. By incorporating the discussed techniques into your PHP applications, you not only safeguard sensitive data but also build trust with your users. The examples provided are foundational but essential steps toward enhancing your web security posture.
For detailed assistance in implementing robust security measures in your web solutions, consider reaching out to semalt.tools. Our team excels in Web Design and Software Development, ensuring your application remains secure and reliable.
Additional Resources
For further reading on PHP encryption best practices, consider checking the following resources:
- PHP Official Documentation on OpenSSL
- OWASP Cryptographic Storage Cheat Sheet
- Cryptography Documentation